Unity:ログの出力(ファイル編)
目次
はじめに
前回に引き続き、ログの出力(ファイル編)を記述しようと思います。ログファイルへの出力についてですが、C#でいえば log4net / NLog など色々なライブラリがありますが、Monoでは扱いにくいので自力で実装してみます。
Unityを知らない方は、ぜひ こちらの記事 をご参照ください。
ログ出力の関連記事
ソースコード
Unity では標準でログの仕組み(ILogger のインターフェース)を持っており、Loggerの仕組みを準備してくれていますので、今回はそれを使います。
using System;
using System.IO;
using System.Text;
using UnityEngine;
public class FileAppender : ILogHandler
{
public static ILogger Create(string logFile, bool outputConsole)
{
var logger = new FileAppender(logFile, outputConsole);
return new Logger(logger);
}
StreamWriter writer;
bool outputConsole;
private FileAppender(string logFile, bool outputConsole)
{
this.writer = File.AppendText(logFile);
this.outputConsole = outputConsole;
}
public void Close()
{
this.writer.Close();
}
public void LogFormat(LogType logType, UnityEngine.Object context, string format, params object[] args)
{
if (this.outputConsole)
Debug.unityLogger.LogFormat(logType, context, format, args);
StringBuilder sb = new StringBuilder();
sb.Append(DateTime.Now.ToString("yyyy/MM/dd HH:mm:ss.fff"));
sb.Append(" [");
sb.Append(logType.ToString());
sb.Append("] ");
sb.Append(string.Format(format, args));
this.writer.WriteLine(sb.ToString());
}
public void LogException(Exception exception, UnityEngine.Object context)
{
if (this.outputConsole)
Debug.unityLogger.LogException(exception, context);
StringBuilder sb = new StringBuilder();
sb.Append(DateTime.Now.ToString("yyyy/MM/dd HH:mm:ss.fff"));
sb.Append(" [");
sb.Append(LogType.Exception.ToString());
sb.Append("] ");
sb.Append(exception.Message);
sb.AppendLine();
sb.Append(exception.StackTrace);
this.writer.WriteLine(sb.ToString());
}
}
使い方
- フィールドで、ILogger を定義してください。
- Start() で、FileAppenderのインスタンスを作成します。
- OnDestroy()で、FileAppender のClose() メソッドを呼び出します。
- 好きなタイミングで、logger.Log などで出力を行ってください。
ソースコード
- 第1引数:ファイルパス
- 第2引数:Debug.Log へのリダイレクトをします
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class LogTest : MonoBehaviour
{
ILogger logger;
void Start()
{
this.logger = FileAppender.Create("logfile.txt", true);
}
void OnDestroy()
{
if (this.logger.logHandler is FileAppender)
((FileAppender)this.logger.logHandler).Close();
}
void Update()
{
// Infoでログを出力
this.logger.Log("output info!");
try
{
throw new Exception("Exception Fire!!");
}
catch (Exception ex)
{
// Exception でのログ出力
this.logger.LogException(ex);
}
}
}
実行結果(画面)

実行結果(ログファイル)
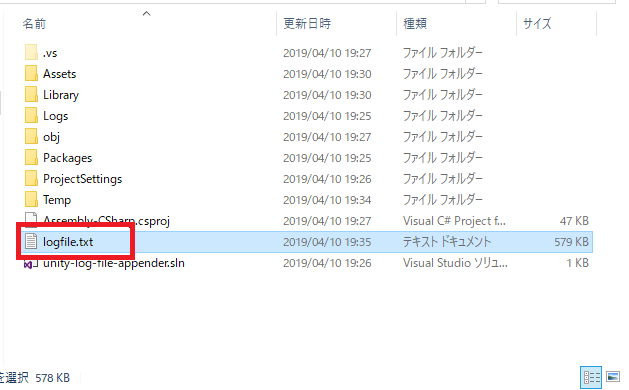
以下のような感じでログが出力されます。
2019/04/10 19:34:25.566 [Log] output info!
2019/04/10 19:34:25.575 [Exception] Exception Fire!!
at LogTest.Update () [0x00013] in C:\Git\fastsystem\unity-log-file-appender\Assets\LogTest.cs:28
2019/04/10 19:34:25.578 [Log] output info!
2019/04/10 19:34:25.579 [Exception] Exception Fire!!
at LogTest.Update () [0x00013] in C:\Git\fastsystem\unity-log-file-appender\Assets\LogTest.cs:28
2019/04/10 19:34:25.833 [Log] output info!
2019/04/10 19:34:25.833 [Exception] Exception Fire!!
at LogTest.Update () [0x00013] in C:\Git\fastsystem\unity-log-file-appender\Assets\LogTest.cs:28
関連リンク
よければ、SNSにシェアをお願いします!